- May 11, 2019
- admin
- 0
API Automation
Here are the steps to follow to automate Rest API Automation..
1. Right click on Solution name, click on “Manage NuGetPackages…”
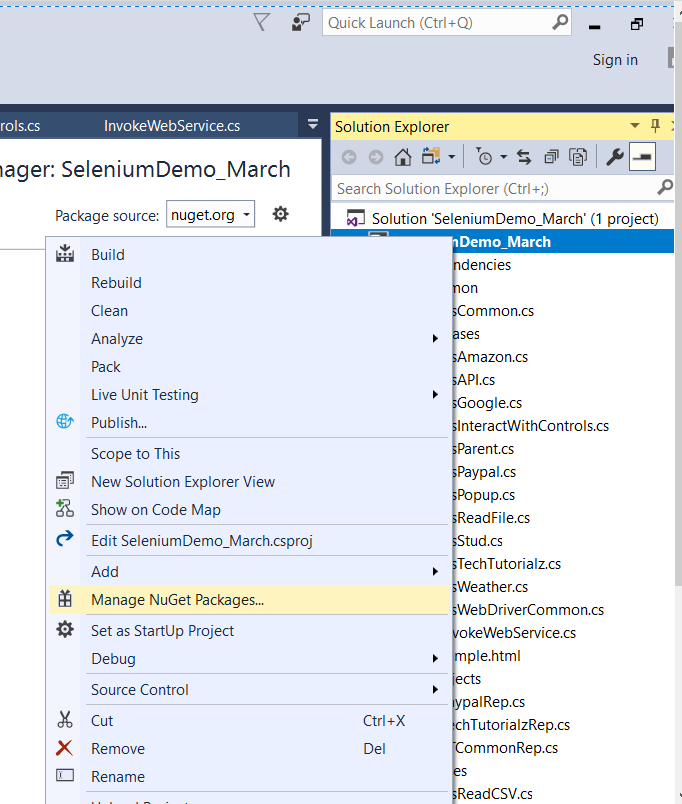
2. Search for “RestSharp”
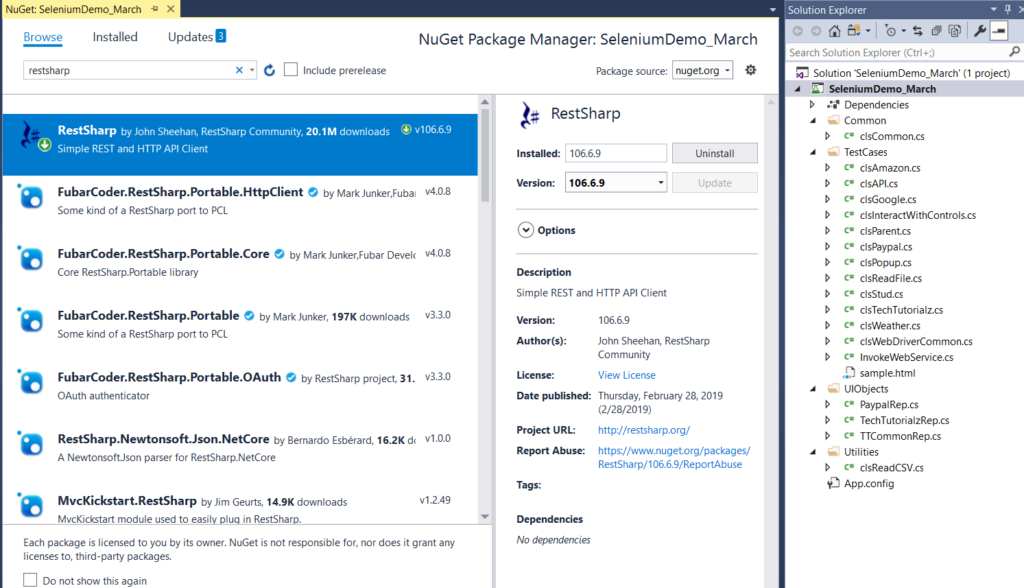
3. Click on “Install” button to install “RestSharp” package
4. Create a class file “clsAPI.cs”
5. Create a class file “clsCommon.cs” to have the common methods.
using System; using System.Collections.Generic; using System.Text; using NUnit; using NUnit.Framework; using RestSharp; using SeleniumDemo_March.Common; namespace SeleniumDemo_March.TestCases { public class clsAPI { clsCommon objCommon = new clsCommon(); [Test] public void StatusCodeTest() { try { //http://restapi.demoqa.com/utilities/weather/city/Hyderabad // arrange RestClient client = new RestClient("http://restapi.demoqa.com"); RestRequest request = new RestRequest("utilities/weather/city/Hyderabad", Method.GET); // act IRestResponse response = client.Execute(request); // assert Assert.That(response.StatusCode, Is.EqualTo(System.Net.HttpStatusCode.OK)); Console.WriteLine(response.Content); Assert.IsTrue(response.Content.Contains("Bangalore") == true); //Assert.IsTrue(response.Content.Contains("Bangalore") == true); } catch(Exception ex) { Console.WriteLine(ex.Message); } } [Test] public void verifyAPIResponse() { string apiResponse= objCommon.GetAPIResponse("http://restapi.demoqa.com", "/utilities/weather/city/Hyderabad"); string apiStatuscode = objCommon.GetAPIStatus("http://restapi.demoqa.com", "/utilities/weather/city/Hyderabad"); if (apiResponse!=null && apiStatuscode == "OK") { Assert.IsTrue(apiResponse.Contains("Hyderabad") == true); } else { Console.WriteLine("API Status code: " + apiStatuscode); Assert.Fail(); } } [Test] public void verifyAPIStatusCode() { string apiStatuscode = objCommon.GetAPIStatus("http://restapi.demoqa.com", "/utilities/weather/city/Hyderabad"); if (apiStatuscode == "OK") { Console.WriteLine("API is working is fine"); } else { Console.WriteLine("API Status code: " + apiStatuscode); Assert.Fail(); } } } }
using System; using System.Collections.Generic; using System.Text; using OpenQA.Selenium; using RestSharp; namespace SeleniumDemo_March.Common { public class clsCommon { public string GetAPIResponse(string ApiEndpoint,string ApiParams) { // arrange RestClient client = new RestClient(ApiEndpoint); RestRequest request = new RestRequest(ApiParams, Method.GET); IRestResponse response = client.Execute(request); Console.WriteLine(response.Content); return response.Content; } public string GetAPIStatus(string ApiEndpoint, string ApiParams) { RestClient client = new RestClient(ApiEndpoint); RestRequest request = new RestRequest(ApiParams, Method.GET); IRestResponse response = client.Execute(request); return response.StatusCode.ToString(); } } }
Learn more about API testing.